Easy methods to Retrieve the IDs, Titles, and URLs of the Core WordPress/WooCommerce Pages
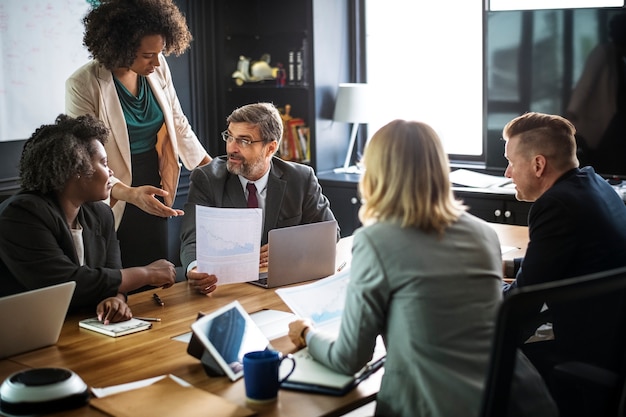
As WordPress builders, many instances we need to seize totally different data (ID, title, URL) for normal WordPress/WooCommerce pages just like the Weblog and My Account pages.
Surprisingly, all this handy stuff is saved within the *_options desk, and we are able to entry it utilizing the get_option() operate. There’s additionally the wp_load_alloptions() that we are able to use to print all of them on the web page.
Let’s see it in motion!
Get Static WordPress Pages
Think about that, on our web site, we’ve registered static pages as entrance and weblog pages.
WordPress shops the ID of those pages as values of the page_on_front and page_for_posts choices.
From there we are able to entry the titles and URLs utilizing the built-in get_the_title() and get_permalink() capabilities.
It’s price noting that one other means of instantly getting the weblog archive web page URL is through the use of the get_post_type_archive_link() operate and passing the put up key phrase as a parameter. For instance, if we need to seize the archive web page URL for the member customized put up kind, we’ll cross the member parameter to this operate.
Static Entrance Web page
1 | /* GET URL */ |
2 | operate get_front_page_url() { |
3 | return get_permalink( get_option( ‘page_on_front’ ) ); |
4 | } |
5 | |
6 | /* GET TITLE */ |
7 | operate get_front_page_title() { |
8 | return get_the_title( get_option( ‘page_on_front’ ) ); |
9 | } |
Static Weblog Web page
1 | /* GET URL */ |
2 | // Technique 1 |
3 | operate get_blog_page_url() { |
4 | return get_permalink( get_option( ‘page_for_posts’ ) ); |
5 | } |
6 | |
7 | // Technique 2 – name get_blog_page_url2( ‘put up’ ) |
8 | operate get_blog_page_url2( $kind ) { |
9 | return get_post_type_archive_link( $kind ); |
10 | } |
11 | |
12 | /* GET TITLE */ |
13 | operate get_blog_page_title() { |
14 | return get_the_title( get_option( ‘page_for_posts’ ) ); |
15 | } |
Get Static WooCommerce Pages
Amongst others, WooCommerce additionally shops the IDs of its important pages like My Account, Store, Cart, and Checkout pages within the *_options desk.
As mentioned above, we are able to then entry their titles and URLs utilizing WordPress core capabilities.
WooCommerce additionally supplies some helpful capabilities like those beneath that we are able to use to entry immediately the web page components we wish:
My Account Web page
1 | /* GET URL */ |
2 | // Technique 1 |
3 | operate get_my_account_page_url() { |
4 | return get_permalink( get_option( ‘woocommerce_myaccount_page_id’ ) ); |
5 | } |
6 | |
7 | // Technique 2 |
8 | operate get_my_account_page_url2() { |
9 | return get_permalink( wc_get_page_id( ‘myaccount’ ) ); |
10 | } |
11 | |
12 | // Technique 3 |
13 | operate get_my_account_page_url3() { |
14 | return wc_get_page_permalink( ‘myaccount’ ); |
15 | } |
16 | |
17 | /* GET TITLE */ |
18 | // Technique 1 |
19 | operate get_my_account_page_title() { |
20 | return get_the_title( get_option( ‘woocommerce_myaccount_page_id’ ) ); |
21 | } |
22 | |
23 | // Technique 2 |
24 | operate get_my_account_page_title2() { |
25 | return get_the_title( wc_get_page_id( ‘myaccount’ ) ); |
26 | } |
Store Web page
1 | /* GET URL */ |
2 | // Technique 1 |
3 | operate get_shop_page_url() { |
4 | return get_permalink( get_option( ‘woocommerce_shop_page_id’ ) ); |
5 | } |
6 | |
7 | // Technique 2 |
8 | operate get_shop_page_url2() { |
9 | return get_permalink( wc_get_page_id( ‘store’ ) ); |
10 | } |
11 | |
12 | // Technique 3 |
13 | operate get_shop_page_url3() { |
14 | return wc_get_page_permalink( ‘store’ ); |
15 | } |
16 | |
17 | /* GET TITLE */ |
18 | // Technique 1 |
19 | operate get_shop_page_title() { |
20 | return get_the_title( get_option( ‘woocommerce_shop_page_id’ ) ); |
21 | } |
22 | |
23 | // Technique 2 |
24 | operate get_shop_page_title2() { |
25 | return get_the_title( wc_get_page_id( ‘store’ ) ); |
26 | } |
Cart Web page
1 | /* GET URL */ |
2 | // Technique 1 |
3 | operate get_cart_page_url() { |
4 | return get_permalink( get_option( ‘woocommerce_cart_page_id’ ) ); |
5 | } |
6 | |
7 | // Technique 2 |
8 | operate get_cart_page_url2() { |
9 | return get_permalink( wc_get_page_id( ‘cart’ ) ); |
10 | } |
11 | |
12 | // Technique 3 |
13 | operate get_cart_page_url3() { |
14 | return wc_get_page_permalink( ‘cart’ ); |
15 | } |
16 | |
17 | // Technique 4 |
18 | operate get_cart_page_url4() { |
19 | return wc_get_cart_url(); |
20 | } |
21 | |
22 | /* GET TITLE */ |
23 | // Technique 1 |
24 | operate get_cart_page_title() { |
25 | return get_the_title( get_option( ‘woocommerce_cart_page_id’ ) ); |
26 | } |
27 | |
28 | // Technique 2 |
29 | operate get_cart_page_title2() { |
30 | return get_the_title( wc_get_page_id( ‘cart’ ) ); |
31 | } |
Checkout Web page
1 | /* GET URL */ |
2 | // Technique 1 |
3 | operate get_checkout_page_url() { |
4 | return get_permalink( get_option( ‘woocommerce_checkout_page_id’ ) ); |
5 | } |
6 | |
7 | // Technique 2 |
8 | operate get_checkout_page_url2() { |
9 | return get_permalink( wc_get_page_id( ‘checkout’ ) ); |
10 | } |
11 | |
12 | // Technique 3 |
13 | operate get_checkout_page_url3() { |
14 | return wc_get_page_permalink( ‘checkout’ ); |
15 | } |
16 | |
17 | // Technique 4 |
18 | operate get_checkout_page_url4() { |
19 | return wc_get_checkout_url(); |
20 | } |
21 | |
22 | /* GET TITLE */ |
23 | // Technique 1 |
24 | operate get_checkout_page_title() { |
25 | return get_the_title( get_option( ‘woocommerce_checkout_page_id’ ) ); |
26 | } |
27 | |
28 | // Technique 2 |
29 | operate get_checkout_page_title2() { |
30 | return get_the_title( wc_get_page_id( ‘checkout’ ) ); |
31 | } |
Different WooCommerce Plugins
Not surprisingly, different WooCommerce plugins retailer data about their pages within the *_options desk. For instance, think about the Yith WooCommerce Wishlist well-liked plugin that permits customers to save lots of their well-liked merchandise on the Wishlist web page.
So long as we set up and activate it, within the database we’ll see a brand new entry for the Wishlist web page.
1 | /* GET URL */ |
2 | // Technique 1 |
3 | operate get_yith_wishlist_page_url() { |
4 | return get_permalink( get_option( ‘yith_wcwl_wishlist_page_id’ ) ); |
5 | } |
6 | |
7 | // Technique 2 |
8 | operate get_yith_wishlist_page_url2() { |
9 | return YITH_WCWL()->get_wishlist_url(); |
10 | } |
11 | |
12 | /* GET TITLE */ |
13 | operate get_yith_wishlist_page_title() { |
14 | return get_the_title( get_option( ‘yith_wcwl_wishlist_page_id’ ) ); |
15 | } |
Conclusion
Accomplished! Right now, we realized totally different strategies for accessing the IDs, titles, and URLs of the core WordPress and WooCommerce pages. I hope you’ll have this tutorial as a reference any time you need to entry such a web page. Final however not least, all of the code coated right here is included on this Gist.
Keep in mind that the easiest way to be taught and discover what you need is by wanting within the database and checking the native information of WordPress and WooCoomerce.
As at all times, thanks rather a lot for studying!