The right way to Create a 2D Digital Clock with HTML, CSS, and Vanilla JavaScript
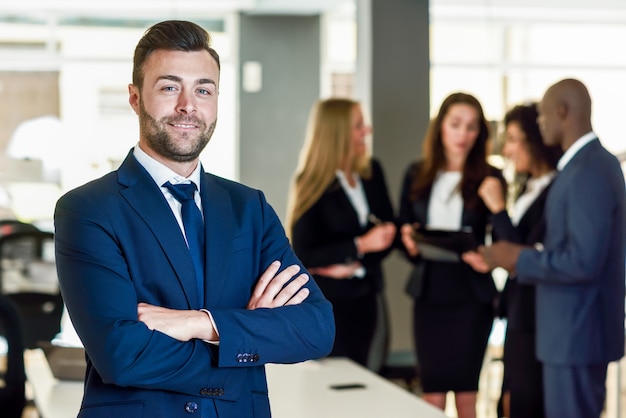
Wouldn’t it’s enjoyable to create your personal stunning Digital Clock with fundamental internet applied sciences? This tutorial will cowl how you can create a faux-3D digital Clock with HTML, CSS, and Vanilla JavaScript.
Let’s get began!
HTML Construction
The HTML construction will encompass an oblong container with a time show inside. The time show will include parts for the present hour, minutes, and seconds.
1 | class=“rectangle-container”> |
2 | class=“rectangle”> |
3 | class=“time”> |
4 | class=“hours”>12 |
5 | class=“separator”>: |
6 | class=“minutes”>00 |
7 | class=“seconds”> |
8 | |
9 | |
10 |
Styling with CSS
First, let’s outline the worldwide types:
1 | :root { |
2 | –background: #dde1e7; |
3 | –rectangle-background: rgb(227, 222, 231); |
4 | –shadow-light: rgba(255, 255, 255, 0.45); |
5 | –shadow-medium: rgba(94, 104, 121, 0.3); |
6 | –shadow-dark:rgba(0, 0, 0, 0.45); |
7 | –darkBlue:rgb(0, 0, 45); |
8 | –time-text-light: #dde1e7; |
9 | –textColor:#fff; |
10 | } |
Then, we’ll model the physique to make sure all our parts are on the heart of the web page.
1 | physique { |
2 | show: flex; |
3 | justify-content: heart; |
4 | align-items: heart; |
5 | min-height: 100vh; |
6 | background: var(–background); |
7 | font-family: “Protest Guerrilla”, sans-serif; |
8 | } |
Fake 3D Impact
The following step is to create an oblong factor to accommodate our digital clock. To make sure the rectangle seems slanted like within the demo, we are going to apply the model rework: skewY(-5deg);. We may also add a field shadow to the correct and the highest and inset field shadows on all sides.
1 | .rectangle { |
2 | place: relative; |
3 | width: 340px; |
4 | top: 120px; |
5 | rework: skewY(-5deg); |
6 | border-bottom-right-radius: 16px; |
7 | box-shadow: inset -5px -5px 9px var(–shadow-light), |
8 | inset 5px 5px 9px var(–shadow-light), |
9 | 0px 10px 10px -10px var(–shadow-dark), |
10 | 10px 0px 10px -10px var(–shadow-dark); |
11 | } |
The following step is to create an phantasm of depth on the left and the highest of the rectangle; we are going to do this by including pseudo-elements to the left and high sides.
Let’s begin with the left facet.
1 | .rectangle::earlier than { |
2 | content material: “”; |
3 | place: absolute; |
4 | high: 0; |
5 | left: -30px; |
6 | width: 30px; |
7 | top: 100%; |
8 | background: var(–background); |
9 | transform-origin: proper; |
10 | rework: skewY(45deg); |
11 | box-shadow: 0px 10px 10px -10px var(–shadow-dark), |
12 | 10px 0px 10px -10px var(–shadow-dark); |
13 | } |
By specifying the peak as 100%, we wish the factor to cowl all the top of the rectangle. By setting rework: skewY(45deg) property, it skews this factor by 45 levels alongside the Y-axis, giving it a slanted look.
The box-shadow property provides a shadow impact to this slanted factor, enhancing its three-dimensional look. You may mess around with the values to realize the specified look.
On this pseudo-element, we additionally add a field shadow on the correct and backside of the factor. The unfold radius of the shadow is ready to a unfavorable worth to make sure that the shadow travels towards the within of the factor.
We’re additionally utilizing absolute positioning to make sure the factor suits precisely on the left facet of the rectangle.
For the highest pseudo-element, we have now the next:
1 | .rectangle::after { |
2 | content material: “”; |
3 | place: absolute; |
4 | high: -30px; |
5 | left: 0; |
6 | width: 100%; |
7 | top: 30px; |
8 | background: rgb(227, 222, 231); |
9 | transform-origin: backside; |
10 | rework: skewx(45deg); |
11 | box-shadow: -5px -5px 9px var(–shadow-light), |
12 | 5px 5px 9px var(–shadow-medium); |
13 | } |
The pseudo-element will stretch all the width of the rectangles and use absolute positioning to make sure it suits exactly on high of the rectangle when skewed. We’re including a field shadow with a minimal blur on all sides of this factor.
At this level, we have now one thing like this:
The Time
Let’s work on the nested time factor, which incorporates the clock.
1 | .time { |
2 | show: flex; |
3 | font-weight: 900; |
4 | justify-content: space-around; |
5 | align-items: heart; |
6 | text-align: heart; |
7 | place: absolute; |
8 | margin: 5px 25px 0 10px; |
9 | width: 300px; |
10 | top: 80px; |
11 | high: 48.5%; |
12 | left: 50%; |
13 | rework: translate(-50%, -50%) skewY(-5deg); |
14 | font-size: 40px; |
15 | transform-origin: proper; |
16 | background-color: clear; |
17 | box-shadow: inset -5px -5px 9px var(–shadow-light), |
18 | inset 5px 5px 9px var(–shadow-medium); |
19 | } |
Use Flexbox to make sure the kid parts are aligned on the heart and distributed evenly contained in the father or mother. Set the font to daring and specify a width and top to make sure it doesn’t overflow outdoors the rectangle.
We’re additionally skewing it to make sure the content material flows with the form of the enclosing factor. The inset box-shadow on all sides creates an phantasm of depth.
The ultimate piece is to model the content material .
1 | .hours, |
2 | .separator, |
3 | .minutes, |
4 | .seconds { |
5 | background: var(–darkBlue); |
6 | shade: var(–textColor); |
7 | margin-left: 10px; |
8 | padding: 2px 10px; |
9 | border-radius: 10px; |
10 | font-weight: 900; |
11 | } |
12 | .seconds { |
13 | font-size: 15px; |
14 | padding: 10px 4px; |
15 | |
16 | } |
By doing this final step we have now utilized some margin and padding to make sure sufficient spacing and readability and background shade to make the textual content seen.
JavaScript Performance
Let’s use JavaScript to make the clock useful. Create a perform referred to as updateTime().
1 | perform updateTime() { |
2 | |
3 | }); |
Contained in the perform, Let’s first get all the weather that may have to be up to date.
1 | const hours = doc.querySelector(“.hours“); |
2 | const minutes = doc.querySelector(“.minutes“); |
3 | const seconds = doc.querySelector(“.seconds“); |
Create a variable referred to as currentTime to retailer the present time. The present time is gotten utilizing the brand new Date() constructor, as proven under.
1 | const currentTime = new Date(); |
The built-in Date() object has a number of GET strategies that we are able to use to particular elements akin to the present 12 months, the present month, the present hour, the present minutes, and so forth.
We want the next strategies.
- getHours(): returns the present hour (0-23).
- getMinutes(): returns the present minutes (0-59).
- getSeconds(): returns the present seconds (0-59).
Let’s get the present hour, minutes, and seconds and replace the corresponding parts.
1 | hours.innerHTML = currentTime.getHours().toString().padStart(2, “0“); |
2 | minutes.innerHTML = currentTime.getMinutes().toString().padStart(2, “0“); |
3 | seconds.innerHTML = currentTime.getSeconds().toString().padStart(2, “0“); |
Updating the Time
The clock now reveals the present time, however it’s not updating. To replace it, we are going to use the setInterval(). setInterval() is a built-in technique that updates a perform after a selected time interval; in our case, we wish the UpdateTime() perform to be up to date each 1 second (1000 milliseconds).
1 | setInterval(updateTime, 1000); |
And right here is the ultimate demo!
Conclusion
This tutorial has lined how you can use CSS and JavaScript to construct a sensible faux-3D Digital clock. Hopefully, it will function an inspiration to construct extra superior and interesting elements.