Create a Rotating Textual content Animation Impact With CSS Variables and JavaScript
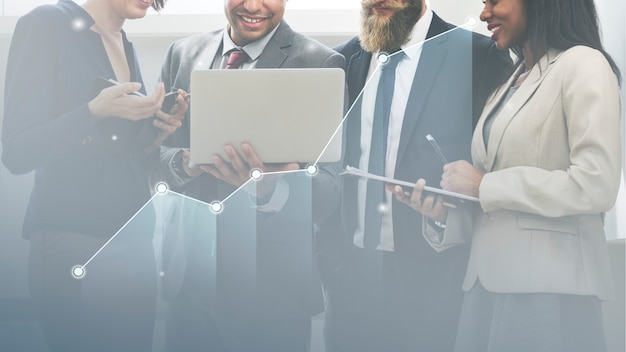
On this new tutorial, we’ll discover ways to create a rotating textual content animation impact utilizing CSS variables and JavaScript. Though we will definitely construct a easy text-changing animation in pure CSS, we’ll put within the loop JavaScript to make issues extra maintainable and efficient.
Fundamental Textual content Rotating Animation
We’ll outline a h1 ingredient the place we’ll put as spans all of the phrases that we wish to animate. By default, all spans may have the data-bg-color and data-color customized attributes that can decide their textual content and background colours accordingly. Plus, initially, solely the span with the present class might be seen—the primary one in our case.
Right here’s the required markup format:
1 | class=“words-wrapper”> |
2 | I wish to be taught class=“css”>CSS and |
3 | class=“phrases”> |
4 | class=“present” data-bg-color=“#ffc703” data-color=“#000”>React |
5 | data-bg-color=“#004e98” data-color=“#fff”>TypeScript |
6 | data-bg-color=“#8cb369” data-color=“#000”>Python |
7 | data-bg-color=“#104911” data-color=“#fff”>PrestaShop |
8 | data-bg-color=“#b8c0ff” data-color=“#000”>Ruby |
9 | data-bg-color=“#e71d36” data-color=“#fff”>Angular |
10 | data-bg-color=“#e2c044” data-color=“#000”>WordPress |
11 | data-bg-color=“#065a82” data-color=“#fff”>Node |
12 | . |
13 |
As we’ve executed in lots of different tutorials prior to now, we’ll use CSS Grid to position all phrases on prime of one another. However keep in mind that every time, solely the phrase with the present class will seem.
Listed below are all of the required kinds:
1 | .words-wrapper { |
2 | font-size: 40px; |
3 | font-weight: daring; |
4 | text-align: heart; |
5 | } |
6 | |
7 | .words-wrapper .css { |
8 | colour: #2ec4b6; |
9 | } |
10 | |
11 | .words-wrapper .phrases { |
12 | show: inline-grid; |
13 | padding: 0 10px; |
14 | border-radius: 6px; |
15 | colour: var(–color, #000); |
16 | background: var(–color-bg, #ffc703); |
17 | } |
18 | |
19 | .words-wrapper .phrases span { |
20 | grid-area: 1/1; |
21 | show: none; |
22 | } |
23 | |
24 | .words-wrapper .phrases span.present { |
25 | show: block; |
26 | } |
To indicate a unique phrase after a sure period of time, we’ll comply with this strategy:
- Each 1.5 seconds, we’ll goal the seen (energetic) phrase.
- Then, we’ll discover its adjoining sibling phrase if it exists, in any other case, we’ll get the primary phrase.
- We’ll take away the present class from the energetic phrase and add it to the brand new ingredient.
- Lastly, we’ll seize the colours of the brand new energetic phrase and replace the corresponding CSS variables.
Right here’s the required JavaScript:
1 | const wrapper = doc.querySelector(“.phrases“); |
2 | const CURRENT_CLASS = “present“; |
3 | |
4 | setInterval(() => { |
5 | const currentWord = wrapper.querySelector(“span.present“); |
6 | const nextWord = currentWord.nextElementSibling |
7 | ? currentWord.nextElementSibling |
8 | : wrapper.firstElementChild; |
9 | currentWord.classList.take away(CURRENT_CLASS); |
10 | nextWord.classList.add(CURRENT_CLASS); |
11 | wrapper.fashion.setProperty(“–color“, nextWord.dataset.colour); |
12 | wrapper.fashion.setProperty(“–color-bg“, nextWord.dataset.bgColor); |
13 | }, 1500); |
We’ll find yourself with the next demo:
Complicated Textual content Rotating Animation
Let’s now construct on the earlier instance and create one thing extra elegant!
The markup will stay the identical aside from one change; this time, we’ll add the following class to the phrase that comes after the energetic one, like this:
1 | class=“words-wrapper”> |
2 | I wish to be taught class=“css”>CSS and |
3 | class=“phrases”> |
4 | class=“present” data-bg-color=“#ffc703” data-color=“#000”>React |
5 | class=“subsequent” data-bg-color=“#004e98” data-color=“#fff”>TypeScript |
6 | data-bg-color=“#8cb369” data-color=“#000”>Python |
7 | data-bg-color=“#104911” data-color=“#fff”>PrestaShop |
8 | data-bg-color=“#b8c0ff” data-color=“#000”>Ruby |
9 | data-bg-color=“#e71d36” data-color=“#fff”>Angular |
10 | data-bg-color=“#e2c044” data-color=“#000”>WordPress |
11 | data-bg-color=“#065a82” data-color=“#fff”>Node |
12 | . |
13 |
Not like the earlier instance, we received’t use CSS Grid to place the phrases, however as an alternative, every phrase might be an absolute positioned ingredient. Their fast dad or mum (i.e. the .phrases ingredient) may have a width that can rely upon the width of the energetic phrase. The subsequent phrase will seem easily from backside to prime after which transfer to the highest to vanish.
On small screens the place the textual content splits into multiple line, to keep away from line flickering which will happen relying on the energetic phrase’s width, we’ll set all phrases’ widths equal to the width of the biggest phrase. On this case, we’ll use the !essential key phrase to override the width of the .phrases ingredient that is set through the width CSS variable.
Listed below are all of the required kinds:
1 | .words-wrapper { |
2 | font-size: 40px; |
3 | font-weight: daring; |
4 | text-align: heart; |
5 | } |
6 | |
7 | .words-wrapper .css { |
8 | colour: #2ec4b6; |
9 | } |
10 | |
11 | .words-wrapper .phrases { |
12 | show: inline-block; |
13 | place: relative; |
14 | vertical-align: backside; |
15 | width: var(–width); |
16 | peak: 60px; |
17 | padding: 0 10px; |
18 | border-radius: 6px; |
19 | colour: var(–color, #000); |
20 | background: var(–color-bg, #ffc703); |
21 | box-sizing: content-box; |
22 | transition: all 0.7s; |
23 | } |
24 | |
25 | .words-wrapper .phrases span { |
26 | place: absolute; |
27 | prime: 0; |
28 | left: 50%; |
29 | opacity: 0; |
30 | remodel: translate(-50%, -100%); |
31 | transition: remodel 0.7s, opacity 0.25s 0.25s; |
32 | } |
33 | |
34 | .words-wrapper .phrases span.present { |
35 | opacity: 1; |
36 | remodel: translate(-50%, 0); |
37 | } |
38 | |
39 | .words-wrapper .phrases span.subsequent { |
40 | remodel: translate(-50%, 100%); |
41 | } |
42 | |
43 | @media (max-width: 700px) { |
44 | .words-wrapper .phrases { |
45 | width: var(–width-mobile) !essential; |
46 | } |
47 | } |
When the DOM is prepared, we’ll specify the preliminary width of the .phrases wrapper by updating the values of the width and width-mobile CSS variable values.
Subsequent, just like the earlier instance, to point out a unique phrase after a sure period of time, we’ll comply with this strategy:
- Each 1.5 seconds, we’ll goal the seen (energetic) and subsequent energetic phrases.
- Then, we’ll discover the adjoining sibling phrase of the following energetic phrase if it exists, in any other case, we’ll get the primary phrase.
- We’ll take away the present class from the energetic phrase and the following class from the following energetic phrase.
- We’ll add the present class to the following energetic phrase and the following class to its adjoining sibling phrase.
- Lastly, we’ll seize the width and colours of the brand new energetic phrase and replace the corresponding CSS variables.
Right here’s the required JavaScript:
1 | const wrapper = doc.querySelector(“.phrases“); |
2 | const phrases = wrapper.querySelectorAll(“span“); |
3 | const currentWord = wrapper.querySelector(“span.present“); |
4 | const wordsWidths = Array.from(phrases).map((phrase) => phrase.offsetWidth); |
5 | const maxWordsWidth = Math.max(…wordsWidths); |
6 | const CURRENT_CLASS = “present“; |
7 | const NEXT_CLASS = “subsequent“; |
8 | |
9 | wrapper.fashion.setProperty(“–width“, `${currentWord.offsetWidth}px`); |
10 | wrapper.fashion.setProperty(“–width-mobile“, `${maxWordsWidth}px`); |
11 | |
12 | setInterval(() => { |
13 | const currentWord = wrapper.querySelector(“span.present“); |
14 | const nextWord = wrapper.querySelector(“span.subsequent“); |
15 | const nextNextWord = nextWord.nextElementSibling |
16 | ? nextWord.nextElementSibling |
17 | : wrapper.firstElementChild; |
18 | currentWord.classList.take away(CURRENT_CLASS); |
19 | nextWord.classList.take away(NEXT_CLASS); |
20 | nextWord.classList.add(CURRENT_CLASS); |
21 | nextNextWord.classList.add(NEXT_CLASS); |
22 | wrapper.fashion.setProperty(“–color“, nextWord.dataset.colour); |
23 | wrapper.fashion.setProperty(“–color-bg“, nextWord.dataset.bgColor); |
24 | wrapper.fashion.setProperty(“–width“, `${nextWord.offsetWidth}px`); |
25 | }, 1500); |
I like to recommend you utilize the browser console to examine the phrases and see how they behave.
We’ll find yourself with the next demo:
Right here’s what we’re going to create. It’s an awesome addition to portfolio web sites or hero sections to focus on issues.
Conclusion
On this tutorial, we used CSS variables and JavaScript to construct a textual content animation impact the place sure phrases change after a selected interval. This can hopefully encourage you to create much more thrilling issues by altering maybe multiple phrase concurrently, and many others.
As at all times, thanks lots for studying!
Uncover Extra JavaScript-Primarily based Textual content Results
Inquisitive about extra artistic textual content animation results with JavaScript? In that case, take a look at these tutorials: