The way to Create an Increasing Search Enter with HTML, CSS, and JavaScript
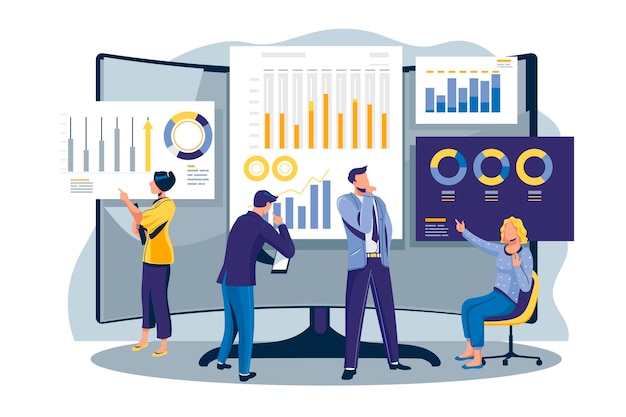
As a designer, you possibly can improve the consumer expertise of a web site or cellular utility by including refined animations to its parts. One such part is a search enter field. This tutorial will cowl the right way to create an increasing search enter; one which expands when a consumer clicks on a search icon.
You’ll discover this kind of increasing search enter impact if ever you employ Youtube within the browser:
HTML Construction
The HTML Construction will likely be only a easy div containing an enter component and a button.
1 | class=“search-wrapper”> |
2 | |
3 | |
4 | id=“search-icon” class=“fa-solid fa-magnifying-glass”> |
5 | |
6 |
You’ll discover that is an enter with a sort of search, not textual content. There’s no actual distinction between the 2, however search is semantically appropriate in our case.
“ components of kind search are textual content fields designed for the consumer to enter search queries into. These are functionally equivalent to textual content inputs, however could also be styled in a different way by the consumer agent.” — mdn
Styling With CSS
The very first thing we need to do is to middle all the pieces utilizing Flexbox. Apply the next kinds to the physique.
1 | physique { |
2 | show: flex; |
3 | align-items: middle; |
4 | justify-content: middle; |
5 | background-color: grey; |
6 | min-height: 100vh; |
7 | } |
Subsequent, we are going to model the complete search enter and later we are going to work on the increasing characteristic. For the wrapper component, add an top, width, border, border-radius and a background .
1 | .search-wrapper { |
2 | width: 50px; |
3 | top: 50px; |
4 | show: flex; |
5 | border-radius: 4rem; |
6 | background: #fff; |
7 | align-items: middle; |
8 | justify-content: middle; |
9 | define: none; |
10 | border: 2px strong #888; |
11 | |
12 | } |
Now we have additionally added the model show: flex to the wrapper div component in order that the kid components .i.e. enter and button may even be centered. For the enter component, set border:none, and description:none. We additionally need it to have a full width and top. Set a margin-left:20px to the placeholder textual content and a few padding.
1 | .search-wrapper enter { |
2 | width: 100%; |
3 | top: 100%; |
4 | padding: 10px; |
5 | margin-left: 20px; |
6 | define: none; |
7 | border: none; |
8 | font-size: 1.5rem; |
9 | |
10 | } |
To make the icon seen, set a background shade to the button. Around the button with border-radius:50%, and place it to the correct of the wrapper component by setting margin-left: auto . The kinds will seem like this:
1 | .search-btn { |
2 | font-size: 2rem; |
3 | border-radius: 50%; |
4 | padding: 10px; |
5 | border: 2px strong white; |
6 | width: 60px; |
7 | cursor: pointer; |
8 | top: 60px; |
9 | background: #e66610; |
10 | margin-left: auto; |
11 | } |
Increasing Characteristic
Now that the complete search enter is full, we will work on the develop characteristic. The very first thing we need to do is cut back the width of the wrapper component.
1 | .search-wrapper { |
2 | width: 60px; |
3 | top: 60px; |
4 | show: flex; |
5 | border-radius: 4rem; |
6 | background: white; |
7 | align-items: middle; |
8 | justify-content: middle; |
9 | border-radius: 100px; |
10 | define: none; |
11 | |
12 | |
13 | } |
Subsequent, Set place:relative to the wrapper div component and place:absolute to the enter component; This may make sure that the enter’s place is relative to the dad or mum component moderately than the physique.
1 | .search-wrapper { |
2 | /* the remainder of the CSS */ |
3 | place: relative; |
4 | } |
5 | |
6 | |
7 | .search-wrapper enter { |
8 | place: absolute; |
9 | high: 0; |
10 | left: 0; |
11 | /* the remainder of the CSS */ |
12 | } |
Now now we have one thing like this.
As you possibly can see, we nonetheless want extra CSS.
Let’s make the button float on high of the enter by setting z-index:1 to the button.
1 | .search-btn { |
2 | font-size: 2rem; |
3 | border-radius: 50%; |
4 | padding: 10px; |
5 | border: 1px strong #be0671; |
6 | width: 60px; |
7 | cursor: pointer; |
8 | top: 60px; |
9 | background: #be0671; |
10 | margin-left: auto; |
11 | z-index: 1; |
12 | |
13 | } |
The ultimate model is to cover any overflow by setting overflow: hidden on the wrapper div component.
1 | .search-wrapper { |
2 | /* remainder of the CSS */ |
3 | overflow: hidden; |
4 | |
5 | } |
JavaScript Performance
The JavaScript performance will develop the wrapper component to full width while you hover with a mouse and cut back the width in case you take away the mouse. Let’s first outline the CSS class for growing the width.
1 | .energetic { |
2 | width: 350px; |
3 | } |
Choose the wrapper div component
1 | const search = doc.querySelector(“.search-wrapper”); |
Subsequent, add two occasion listeners, mouseover and mouseout. On mouseover, we are going to add the energetic CSS model; on mouseout, we are going to take away the CSS model.
1 | search.addEventListener(“mouseover“, () => { |
2 | if (!search.classList.comprises(“energetic“)) { |
3 | search.classList.add(“energetic“); |
4 | } |
5 | }); |
6 | |
7 | search.addEventListener(“mouseout“, () => { |
8 | if (search.classList.comprises(“energetic“)) { |
9 | search.classList.take away(“energetic“); |
10 | } |
11 | }); |
Animation
The ultimate step is so as to add a transition impact to make the transition impact easy.
1 | .search-wrapper { |
2 | /* the remainder of the CSS */ |
3 | transition: 400ms ease-in-out; |
4 | |
5 | } |
You may mess around with the transition values to realize the specified really feel. That’s it for this tutorial; try the CodePen beneath.
Conclusion
This tutorial has coated creating an increasing search bar that features animations to enhance interactivity. Incorporating transitions in your design is important to make the consumer interface extra participating and satisfying.