The way to Create Animated Snow on a Web site (with CSS and JavaScript)
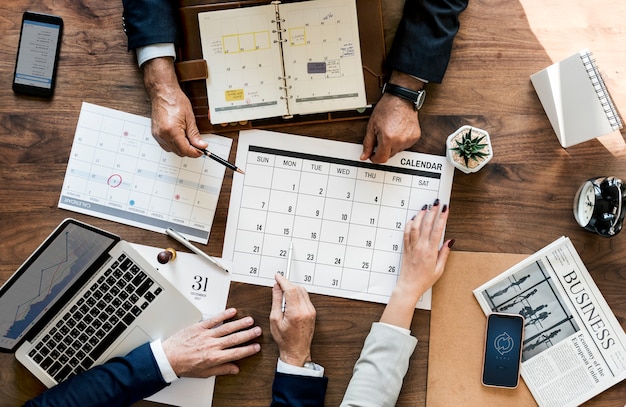
On this tutorial, we’re going to implement an animated snow impact on a webpage utilizing CSS and vanilla JavaScript, simply in time for the vacation season.
‘Tis the season to be jolly so let it snow, let it snow, let it snow!
1. Markup with Vacation HTML
For our markup, we’ll have two major containers: a important component containing the content material of our web page and a snow-container component that can show the snow.
1 | |
2 | Pleased Holidays! |
3 | |
4 | id=“snow-container”> |
5 |
2. Season’s Styling with CSS
On this demo, our important content material has a gradient background color to provide it an evening sky impact. We obtain this utilizing the CSS linear-gradient operate.
For simplicity, let’s say we’re constructing this in CodePen, so the markup goes within the HTML tab, the kinds within the CSS tab, and so forth.
1 | important { |
2 | background: linear-gradient(to backside, #2d91c2 0%, #1e528e 100%); |
3 | show: flex; |
4 | align-items: heart; |
5 | justify-content: heart; |
6 | font-family: “Pacifico”, cursive; |
7 | top: 100vh; |
8 | padding: 20px; |
9 | text-align: heart; |
10 | } |
11 | |
12 | h1 { |
13 | colour: white; |
14 | } |
Right here’s what our banner appears like now:
We’ll additionally use CSS to deal with the animation of every snow component. Every snowflake may have two animations: a fall animation to deal with the snowflake shifting from the highest to the underside of the display screen and a sway animation to deal with shifting the snowflake slowly facet to facet.
1 | @keyframes fall { |
2 | 0% { |
3 | opacity: 0; |
4 | } |
5 | 50% { |
6 | opacity: 1; |
7 | } |
8 | 100% { |
9 | high: 100vh; |
10 | opacity: 1; |
11 | } |
12 | } |
13 | |
14 | @keyframes sway { |
15 | 0% { |
16 | margin-left: 0; |
17 | } |
18 | 25% { |
19 | margin-left: 50px; |
20 | } |
21 | 50% { |
22 | margin-left: -50px; |
23 | } |
24 | 75% { |
25 | margin-left: 50px; |
26 | } |
27 | 100% { |
28 | margin-left: 0; |
29 | } |
30 | } |
We’ll additionally type our snow container and the snow component we’ll be creating in JavaScript.
1 | #snow-container { |
2 | top: 100vh; |
3 | overflow: hidden; |
4 | place: absolute; |
5 | high: 0; |
6 | transition: opacity 500ms; |
7 | width: 100%; |
8 | } |
9 | |
10 | .snow { |
11 | animation: fall ease-in infinite, sway ease-in-out infinite; |
12 | colour: skyblue; |
13 | place: absolute; |
14 | } |
The animation property on this demo takes three values:
- title: That is the worth of the animation we created beneath keyframes.
- timing: This determines how the animation progresses.
- iteration: That is the variety of instances the animation occurs. We use infinite to always repeat the animation of the snowflakes.
3. Fa la la la la Performance ♬
Now we are able to work on the enjoyable half: making it snow! First, within the JS tab in CodePen, let’s assign a worth to our container component:
1 | const snowContainer = doc.getElementById(“snow-container“); |
For our snowflake content material, we’ll be utilizing HTML symbols (❄ ❅ ❆):
We’ll create a snowContent array to include the image codes
1 | const snowContent = [‘❄‘, ‘❅‘, ‘❆‘] |
With a view to create a falling snow impact, we’ll must randomise the looks and animation of every snowflake.
We’ll use the Math.random() and Math.ground() features to deal with producing the random values for our snow components.
1 | const random = (num) => { |
2 | return Math.ground(Math.random() * num); |
3 | } |
Now we are able to create a operate to generate a random type for every snowflake. We’ll generate a random place, measurement and animation length.
1 | const getRandomStyles = () => { |
2 | const high = random(100); |
3 | const left = random(100); |
4 | const dur = random(10) + 10; |
5 | const measurement = random(25) + 25; |
6 | return ` |
7 | high: –${high}%; |
8 | left: ${left}%; |
9 | font-size: ${measurement}px; |
10 | animation-duration: ${dur}s; |
11 | `; |
12 | } |
For our length and measurement fixed, we add a hard and fast quantity worth to make sure the random generated quantity has a minimal worth of the quantity being added (i.e. the bottom quantity for the animation length of any snowflake is 10s)
For our high fixed, we assign a detrimental worth so the snowflakes begins from any share above the seen display screen.
Subsequent, we’ll deal with appending the snowflakes to the snow container.
1 | const createSnow = (num) => { |
2 | for (var i = num; i > 0; i—) { |
3 | var snow = doc.createElement(“div“); |
4 | snow.className = “snow“; |
5 | snow.type.cssText = getRandomStyles(); |
6 | snow.innerHTML = snowContent[random(2)] |
7 | snowContainer.append(snow); |
8 | } |
9 | } |
On this demo, we use a for loop to create a hard and fast variety of snowflakes and append them to the container. We additionally randomly assign the innerHTML as any of the values in our snowContent array.
Then, we’ll name the createSnow operate as soon as our web page hundreds utilizing the load occasion listener.
1 | window.addEventListener(“load“, () => { |
2 | createSnow(30) |
3 | }); |
And it’s snowing! Click on Rerun to see the animated snow:
Eradicating Snowflakes
We will additionally select so as to add a operate to take away the snowflakes.
1 | const removeSnow = () => { |
2 | snowContainer.type.opacity = “0“; |
3 | setTimeout(() => { |
4 | snowContainer.take away() |
5 | }, 500) |
6 | } |
On this operate, we assign our snowContainer an opacity of 0 to permit it fade out easily after which, after half a second, we take away the container utilizing the .take away() technique.
We will then select to name this operate nevertheless we would like. We will take away the snow when the person clicks wherever on the web page:
1 | window.addEventListener(“click on“, () => { |
2 | removeSnow() |
3 | }); |
Or we are able to embody it in setTimeout() operate so it disappears after a sure period of time:
1 | window.addEventListener(“load”, () => { |
2 | createSnow(30) |
3 | setTimeout(removeSnow, 10000) |
4 | }); |
Switching Out the Snowflakes
Since we’ve constructed this from scratch, we are able to additionally replace the component content material and animation to create different enjoyable results, like floating balloons:
and even animated Nyan Cat:
I hope you loved this animated snow tutorial, and it’s made you are feeling good and festive!