Easy methods to Change Picture on Product Hover in WooCommerce Merchandise Loop
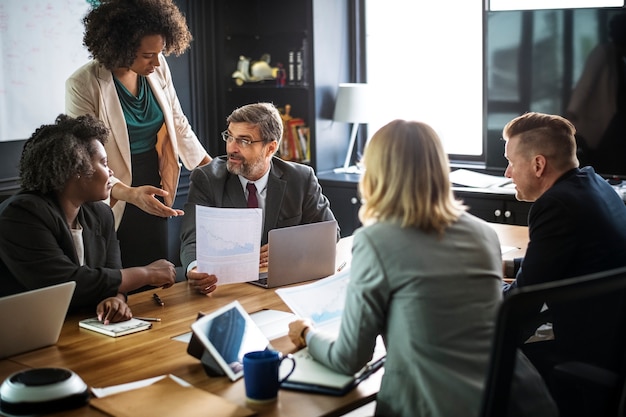
On this tutorial, you’ll learn to add interactivity to your WooCommerce shops by displaying a second picture on product hover inside loops (e.g. archive pages, associated merchandise, and so forth.). This can be a characteristic that many premium WooCommerce themes present. However, as you’ll see, it’s not that arduous to code by ourselves!
This tutorial expects that you’re conversant in WordPress and WooCommerce theme growth.
Right here’s an introductory video that showcases the anticipated habits:
Implementation
As traditional, for higher demonstration, I’ll arrange a customized base theme on a neighborhood WordPress/WooCommerce set up. I’ll work with their newest variations on the time of this writing (WP 6.4.1/WC 8.3.1).
Within the admin, I’ve created three easy merchandise:
Every product has its personal picture and probably a number of further photos—all photos come from Unsplash:
For my design, I would like the product photos to be sq., so I’ll add photos 1000×1000 with the next settings—in your case they could differ:
Subsequent, I’ll disable WooCommerce default types—I all the time do that as I need to have full management of the types:
1 | //features.php |
2 | |
3 | add_filter( ‘woocommerce_enqueue_styles’, ‘__return_empty_array’ ); |
Shifting on, though utterly of secondary significance, I’ll embody my beloved UIkit front-end framework to the theme to hurry up the event course of.
By doing so, my store web page will appear to be this:
Secondary Picture on Hover
This appears okay, however actually, it isn’t the aim of this tutorial. What I would like is a option to change the product picture on hover.
Now, lots of you would possibly marvel which picture will I present. Effectively, we’ve got numerous choices; I can present the primary picture from the product gallery, a random one from that gallery, and even one other picture coming from a picture subject.
On this instance, I’ll go together with the primary possibility and reveal the primary picture from the product gallery. To take action, I’ll override the WooCommerce content-product.php file.
To be extra particular, after the woocommerce_before_shop_loop_item hook, I’ll add a div with the category of image-wrapper which is able to wrap something contained in the woocommerce_before_shop_loop_item_title hook.
This code will try and seize the ID of the primary picture from the product gallery. If it exists, it’ll print its HTML illustration utilizing the wp_get_attachment_image() operate. Be aware that the picture measurement would be the woocommerce_thumbnail one which applies to the catalog pages, and earlier I set it to 750px. Additionally, the picture will obtain the img-back class for simpler concentrating on by the CSS.
Right here’s the required code addition:
1 | /** |
2 | * yourtheme/woocommerce/content-product.php |
3 | * |
4 | * code after the woocommerce_before_shop_loop_item hook |
5 | */ |
6 | |
7 | class=“image-wrapper”> |
8 | |
9 | $first_gal_img_id = reset( $product->get_gallery_image_ids() ); |
10 | |
11 | if ( $first_gal_img_id ) : |
12 | echo wp_get_attachment_image( |
13 | $first_gal_img_id, |
14 | ‘woocommerce_thumbnail’, |
15 | false, |
16 | array( |
17 | ‘class’ => ‘img-back’, |
18 | ) |
19 | ); |
20 | endif; |
21 | |
22 | do_action( ‘woocommerce_before_shop_loop_item_title’ ); |
23 | ?> |
24 |
After overriding this WooCommerce template in my theme, my archive pages will appear to be this:
As you possibly can see, each the primary product picture (backside picture) and the primary product gallery picture (high picture) seem.
What I miss now’s just a few types. Via the CSS, I’ll cover the primary picture and present it solely on hover—relying in your theme, this code won’t work with out tweaks:
1 | .woocommerce-LoopProduct-link { |
2 | show: inline-block; |
3 | } |
4 | |
5 | .woocommerce-LoopProduct-link .image-wrapper { |
6 | place: relative; |
7 | } |
8 | |
9 | .woocommerce-LoopProduct-link .img-back { |
10 | place: absolute; |
11 | high: 0; |
12 | left: 0; |
13 | width: 100%; |
14 | show: none; |
15 | } |
16 | |
17 | /*Optionally available*/ |
18 | .woocommerce-LoopProduct-link:hover { |
19 | text-decoration: none; |
20 | coloration: #333; |
21 | } |
22 | |
23 | .woocommerce-LoopProduct-link:hover .img-back { |
24 | show: block; |
25 | } |
When it comes to the output markup, right here’s the generated HTML for a product contained in the loop:
One other factor to notice is that right here, all my photos have equal dimensions, so I don’t have to make use of properties like object-fit: cowl and peak: 100% to place the second picture.
Overriding WooCommerce Types
Keep in mind that above, I described a situation the place I eliminated WooCommerce default types and began the styling from scratch.
However in case I wished to maintain the default types, I’d change a few of my selectors to match WooCommerce ones and forestall overrides as a result of higher CSS specificity like this:
1 | .woocommerce ul.merchandise li.product a .img-back { |
2 | place: absolute; |
3 | high: 0; |
4 | left: 0; |
5 | width: 100%; |
6 | show: none; |
7 | } |
8 | |
9 | .woocommerce ul.merchandise li.product a:hover .img-back { |
10 | show: block; |
11 | } |
Utilizing Hooks
Another implementation, as a substitute of including the additional code contained in the content-product.php file, is to print this code by two customized features that can run in the course of the woocommerce_before_shop_loop_item_title hook.
An vital facet is to make sure that these features will run within the appropriate order. To make this occur, they need to have particular priorities following the priorities of the remainder of the features of this hook.
With this in thoughts, I’ll place this code within the features.php of my theme:
1 | operate playground_start_wrapper_div_print_second_product_img() { |
2 | world $product; |
3 | echo ‘ ‘; |
4 | $first_gal_img_id = reset( $product->get_gallery_image_ids() ); |
5 | |
6 | if ( $first_gal_img_id ) : |
7 | echo wp_get_attachment_image( |
8 | $first_gal_img_id, |
9 | ‘woocommerce_thumbnail’, |
10 | false, |
11 | array( |
12 | ‘class’ => ‘img-back’, |
13 | ) |
14 | ); |
15 | endif; |
16 | } |
17 | |
18 | operate playground_close_wrapper_div_print_second_product_img() { |
19 | echo ‘ ‘ ; |
20 | } |
21 | |
22 | add_action( ‘woocommerce_before_shop_loop_item_title’, ‘playground_start_wrapper_div_print_second_product_img’, 9 ); |
23 | add_action( ‘woocommerce_before_shop_loop_item_title’, ‘playground_close_wrapper_div_print_second_product_img’, 11 ); |
The types will stay the identical in addition to the top consequence.
Conclusion
Throughout this tutorial, we dived into the WooCommerce e-commerce platform and mentioned two methods to disclose a secondary product picture upon hovering on the merchandise inside loops.
Hopefully, you’ve discovered this train helpful sufficient, and also you’ll give it a shot in your upcoming WooCommerce tasks. Simply keep in mind that relying in your theme setup, particularly in case you haven’t constructed it from scratch, you most likely have to change your code to undertake this performance.
If you wish to see extra WooCommerce suggestions and methods, do tell us by X!
As all the time, thanks loads for studying!