Straightforward Slider (Carousel With Pure CSS)
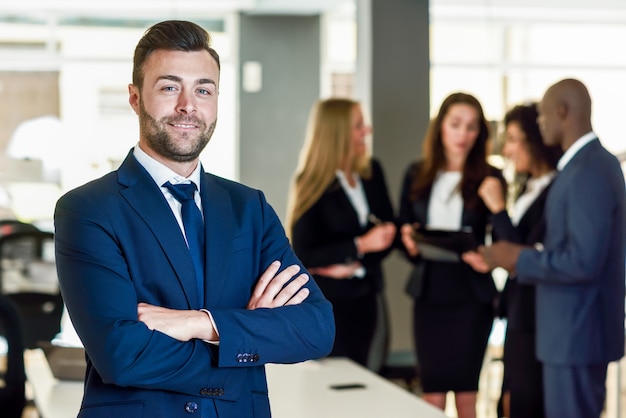
A carousel is a good instance of a attainable CSS-only interactive characteristic. Whereas carousels (aka sliders) are normally constructed with JavaScript, if we take away the complicated options, they’re merely scrollable parts with an overflow; precisely what CSS is made for.
You may be considering “CSS is used for styling whereas JavaScript is used for interactivity, that’s simply the best way the net works.”
Nevertheless, contemplating the truth that CSS is loaded on a webpage sooner than JavaScript, and CSS additionally causes much less reflow on a web page (which improves efficiency), it’s honest to say we must always use CSS for interactivity wherever attainable.
1. Format With HTML
The format of our simple slider is easy: we’ll create a carousel-container div to carry the carousel-slide parts.
1 | class=“carousel-container”> |
2 | class=“carousel-slide”>1 |
3 | … |
4 |
That’s all we’d like so let’s transfer on to the styling
2. Carousel Behaviour with CSS
As soon as we have now our carousel slides arrange, we’ll type the carousel to have the next options:
- Scrollable content material
- Snap to subsequent slide
- Progress bar indicating slide progress
Scrollable Content material
For the scrollable content material, we’ll use the show:flex and overflow-x: auto properties. We’ll additionally type slides so we will see 3 slides on the desktop display screen and 1 slide on cellular.
1 | .carousel-container { |
2 | show: flex; |
3 | overflow-x: auto; |
4 | } |
5 | |
6 | .carousel-slide { |
7 | flex: 1 0 30%; |
8 | } |
9 | |
10 | @media (max-width: 600px) { |
11 | .carousel-slide { |
12 | flex: 1 0 90%; |
13 | } |
14 | } |
Snap to Slide
Subsequent, to attain the snapping impact on the slides, we’ll use the CSS scroll-snap properties. The scroll snap properties permit us outline “snapping” factors on a component (the purpose of the factor that we wish to be totally seen as soon as scrolling). Our up to date code appears to be like like this:
1 | .carousel-container { |
2 | show: flex; |
3 | overflow-x: auto; |
4 | scroll-snap-type: x necessary; |
5 | } |
6 | |
7 | .carousel-slide { |
8 | flex: 1 0 30%; |
9 | scroll-snap-align: middle; |
10 | } |
11 | |
12 | @media (max-width: 600px) { |
13 | .carousel-slide { |
14 | flex: 1 0 90%; |
15 | } |
16 | } |
Elective: CSS-Solely Progress Bar
To maintain inline with our CSS-only interactivity, we will make the most of native browser options to create a progress bar for our carousel. We’ll do that by styling the carousel container scrollbar to offer the looks of a progress bar. That is what the code appears to be like like:
1 | .carousel-container::-webkit-scrollbar { |
2 | top: 8px; |
3 | } |
4 | |
5 | .carousel-container::-webkit-scrollbar-thumb { |
6 | background: #29AB87; |
7 | } |
8 | |
9 | .carousel-container::-webkit-scrollbar-track { |
10 | background: #b1b3b399; |
11 | } |
12 | |
13 | .carousel-container::-webkit-scrollbar-track-piece:begin { |
14 | background: #29AB87; |
15 | } |
Let’s have a look at the properties we’re utilizing:
- ::webkit-scrollbar: the complete scrollbar factor
- ::webkit-scrollbar-thumb: the draggable bar on the scrollbar
- ::webkit-scrollbar-track: the trail that the scrollbar thumb is on
- ::webkit-scrollbar-track-piece:begin: the trail of the monitor not coated by the scrollbar thumb, the :begin selector targets solely the trail behind the scrollbar thumb
Within the diagram above, we will see what elements of the scrollbar are being focused. By making the -webkit-scrollbar-thumb and ::webkit-scrollbar-track-piece:begin the identical color, the scrollbar gives the look of being crammed in because it’s being scrolled, thus making a progress bar.
And since our progress bar is definitely a scrollbar, it may also be used to scroll by way of the carousel as a further characteristic: it’s a win/win!
The ::webkit-scrollbar properties are non-standard, fairly sketchy, and never supported by all browsers so it’s not beneficial to make use of this in a manufacturing surroundings. This implies our progress bar will appear like a daily scrollbar on non-supported browsers, or in the event you select to not embrace these ::webkit-scrollbar guidelines.
That’s all there may be to our simple slider! We have constructed a scrollable container with a nifty snapping characteristic and even added a progress bar utilizing solely CSS:
3. Extra Options With JavaScript
Since we’ve gotten the fundamental characteristic of the carousel out of the best way, we will go on so as to add much more options, utilizing JavaScript this time.
A kind of options is utilizing arrows to deal with the carousel navigation. In a earlier tutorial, we appeared into constructing a carousel with JavaScript (lower than 14 traces of code!), so we will mix that tutorial with this one so as to add much more options to our simple slider.
That is what our mixed carousel appears to be like like:
Only for enjoyable, on this demo the code has been refactored to make use of even fewer traces of JavaScript, so that is what our up to date carousel arrow operate appears to be like like:
1 | const carousel = doc.querySelector(“.carousel-container“); |
2 | const slide = doc.querySelector(“.carousel-slide“); |
3 | |
4 | operate handleCarouselMove(constructive = true) { |
5 | const slideWidth = slide.clientWidth; |
6 | carousel.scrollLeft = constructive ? carousel.scrollLeft + slideWidth : carousel.scrollLeft – slideWidth; |
7 | } |
Then we cross that operate to our HTML arrows:
1 | |
2 | ‹ |
3 | |
4 | |
5 | |
6 | › |
7 |
And with that, we’ll name it a day on our modded-up carousel!